1. Overview
1.1. BunHMI Display
A BunHMI display is shown in Figure 1. It’s base on LVGL and use BunTalk to interact with the GUI widgets. An easy way for you to create GUI applications. Just like the delicious buns. (quick, easy and diverse).
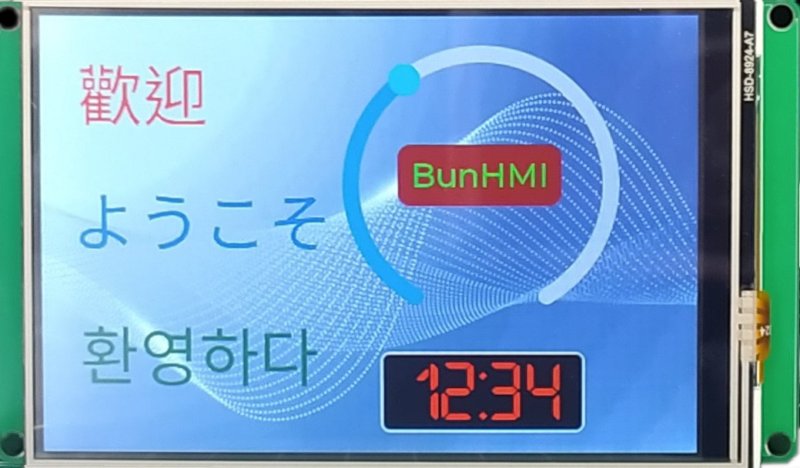
1.2. The Host device
BunHMI use UART to communicate with host which only needs two siganls (Tx,Rx), any host with UART interface can communicate with BunHMI.
Warning
|
The voltage level of BunHMI UART is 3.3V. Which should work fine with 5V TTL interface devices. But not for 5V CMOS devices! |
Warning
|
Don’t connect BunHMI with RS-232 interface. This will cause damage of BunHMI display, and not recoverable. |
The host device maybe an Arduino boards (Figure 2) or MCUs, or even PC via USB-UART(TTL) which can interact with BunHMI easily.
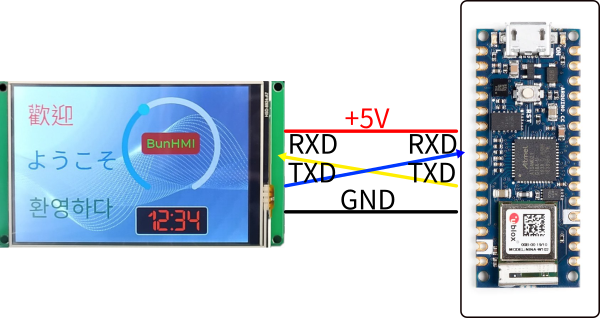
You have to cross Tx & Rx signal between Host and BunHMI display.
-
Host UART Tx → BunHMI Rx
-
Host UART Rx ← BunHMI Tx
The default UART baudrate of BunHMI is: 115200/8n1. You can change the UART baudrate in Project config at BunMaker.
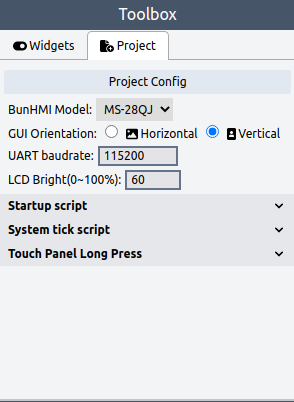
2. BunMaker
BunMaker (Figure 3) is a GUI design app by utilize LVGL. library. BunMaker helps to create LVGL layout.
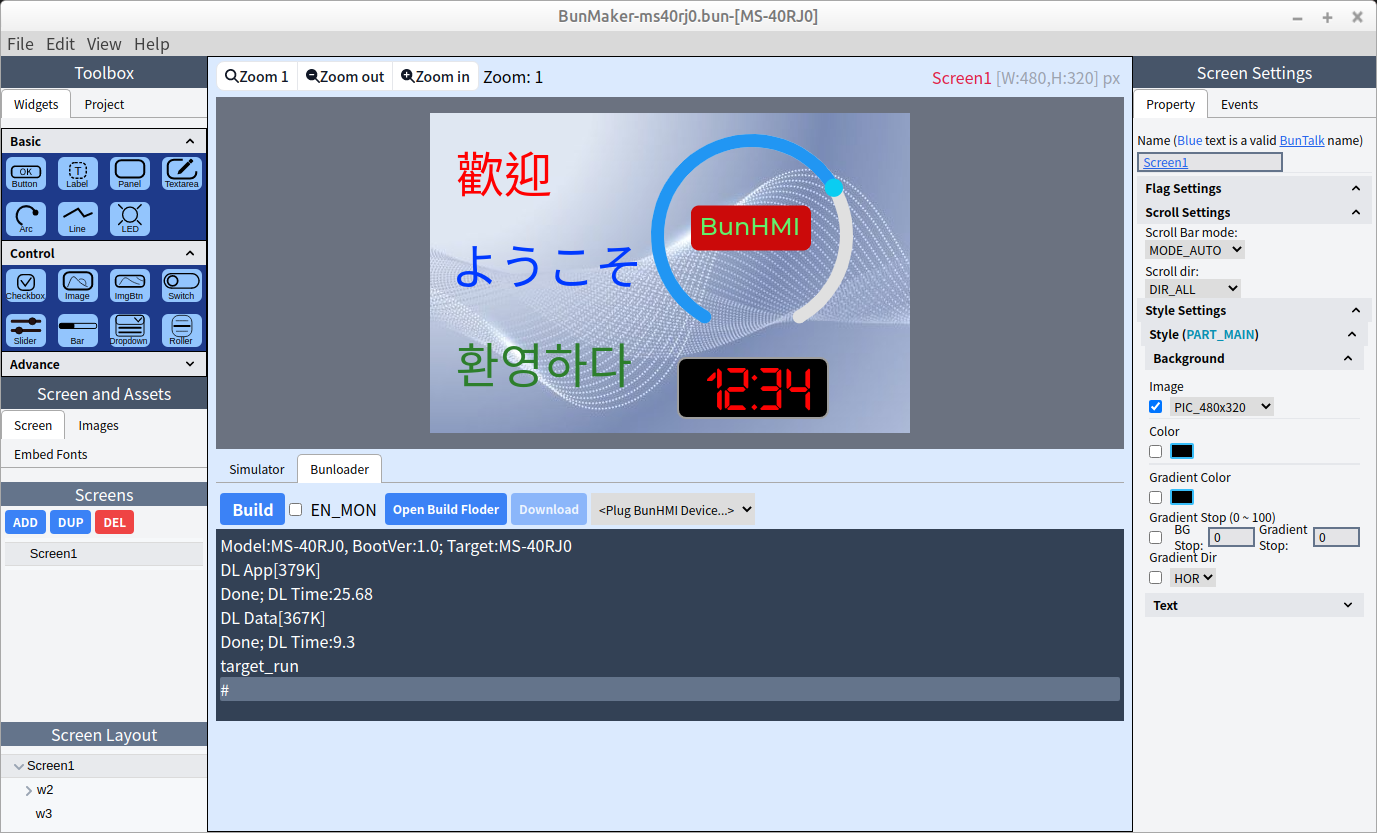
2.1. Widget Naming rule
BunTalk script use widget name to interact with widgets. There is a widget name setting at the Widget Settings Panel (Figure 4) of the right side of BunMaker.
Like most programming code. There is a naming rule. A valid widget name must be
-
Start with char _, a-z, A-Z.
-
And only contain _, a-z, A-Z, 0-9
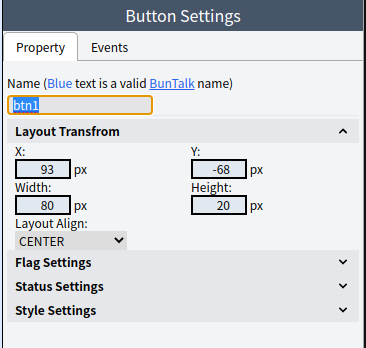
Now you can set the Button property by using BunTalk script like
btn1.width(100); // Set btn1 width to 100px
2.2. Name Scope
In Buntalk the System object "sys" is a global object and always accessable. Other objects includes screen and widget which are created in BunMaker that can be accessed by object name.
- Screens
-
Screens are always accessible, you can access screen name any time.
- Widget Name
-
Widget name is only exist when it’s belong screen is loaded.
You may create multiple screens in one project. like following example.
Screen name | Widget name |
---|---|
Screen1 |
|
Screen2 |
|
And you can load screen by sys.scr() method
sys.scr(Screen1); // load Screen1
//or
sys.scr(Screen2, 1, 200); // load Screen2 with animation type 1 with duration time 200ms
When Screen1 is loaded, you can access following widget by widget name.
-
btn1
-
btn2
-
lab1
You can use Buntalk like
btn1.x(100); // Set btn1 *x* position
lab1.text("Hello"); // Set text display of lab1
When Screen2 is loaded, you can access following widget by widget name.
-
btn1
-
btn2
-
img1
-
img2
The btn1 in Screen1 and Screen2 is different object, depend on which screen is loaded. Also, you can’t access img1 when Screen2 is’t loaded. So, make sure which screen is loaded when access the widget.
A valid name of widget must be an unique name in the same screen.
Tip
|
You can use an invalid widget name like "~background", if you don’t want to interact with that widget. An invalid name do not need be an unique name in the same screen. |
2.3. Widget Event
When interact with GUI, we always interesting in events, and make some response. BunHMI is based on LVGL library. You can write BunTalk script when LVGL events triggered.
Here are the LGVL events:
-
EVENT_CLICKED - An object was pressed for a short period of time, then released. Not called if scrolled
-
EVENT_PRESSED - An object has been pressed
-
EVENT_LONG_PRESSED - An object has been pressed for a longer period of time
-
EVENT_LONG_RRESSED_REPEAT - Called after long_press_time in every long_press_repeat_time ms. Not called if scrolled
-
EVENT_FOCUSED - An object is focused
-
EVENT_DEFOCUSED - An object is unfocused
-
EVENT_VALUE_CHANGED - The value of the object has been changed.
-
EVENT_READY - A process has finished
-
EVENT_CANCEL - A process has been cancelled
-
EVENT_SCREEN_LOADED - A screen was loaded, called when all animations are finished
-
EVENT_SCREEN_UNLOADED - A screen was unloaded, called when all animations are finished
-
EVENT_SCREEN_LOAD_START - A screen load started, fired when the screen change delay is expired
-
EVENT_SCREEN_UNLOAD_START - A screen unload started, fired immediately when
In the widget Settings panel of BunMaker. You can select the Events page. Here list events that relative to the widget. Like figure Figure 5
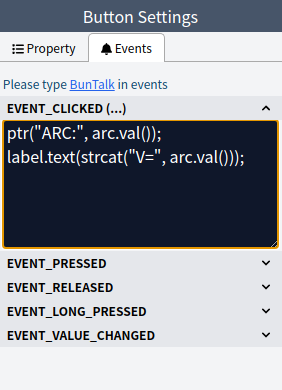
In the above example. if the widget is named "arc" with value=33. When the Button is clicked, BunHMI will run the BunTalk script and print string ARC:33 to UART and concatenate string to V=33 and assign to the widget text which widget is named with "label".
You can write BunTalk script when screen is load, like Figure 6
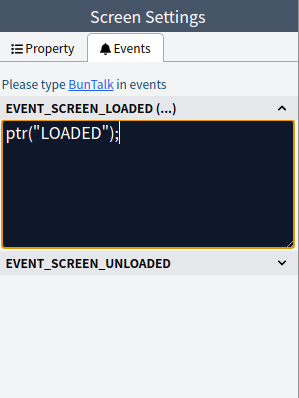
This will send "LOADED" to UART when screen is loaded.
Although BunHMI is base on LVGL, but not all LVGL’s events are supported in BunHMI display. please check the BunMaker’s Widget Events Panel for events that are suppored.
For the detail of events description, please check LVGL Events doc.
2.4. Widget Flags
Widget has flags that will affect its UI behavior. Flag settings are list at Widget settings panel of BunMaker, show as following picture.
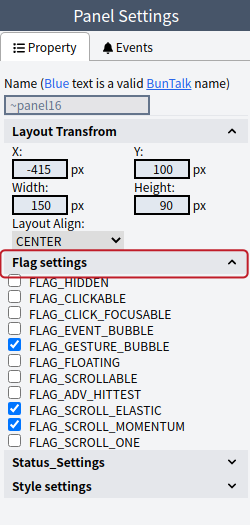
Here are the flags list and description. not all flags applies to each widget.
-
FLAG_HIDDEN - Make the object hidden. (Like it wasn’t there at all)
-
FLAG_CLICKABLE - Make the object clickable by the input devices
-
FLAG_CHECKABLE - Toggle checked state when the object is clicked
-
FLAG_CLICK_FOCUSABLE - Add focused state to the object when clicked
-
FLAG_SCROLLABLE - Make the object scrollable
-
FLAG_SCROLL_ELASTIC - Allow scrolling inside but with slower speed
-
FLAG_SCROLL_MOMENTUM - Make the object scroll further when "thrown"
-
FLAG_SCROLL_ONE - Allow scrolling only one snappable children
-
FLAG_SCROLL_CHAIN_HOR - Allow propagating the horizontal scroll to a parent
-
FLAG_SCROLL_CHAIN_VER - Allow propagating the vertical scroll to a parent
-
FLAG_SCROLL_CHAIN - (FLAG_SCROLL_CHAIN_HOR | FLAG_SCROLL_CHAIN_VER)
-
FLAG_SCROLL_ON_FOCUS - Automatically scroll object to make it visible when focused
-
FLAG_SCROLL_WITH_ARROW - Allow scrolling the focused object with arrow keys
-
FLAG_SNAPPABLE - If scroll snap is enabled on the parent it can snap to this object
-
FLAG_EVENT_BUBBLE - Enabled all events will be sent to an object’s parent too, If the parent also has FLAG_EVENT_BUBBLE enabled the event will be sent to its parent and so on.
-
FLAG_PRESS_LOCK - Keep the object pressed even if the press slid from the object
-
FLAG_EVENT_BUBBLE - Propagate the events to the parent too
-
FLAG_GESTURE_BUBBLE - Propagate the gestures to the parent
-
FLAG_ADV_HITTEST - Allow performing more accurate hit (click) test. E.g. consider rounded corners.
-
FLAG_IGNORE_LAYOUT - Make the object position-able by the layouts
-
FLAG_FLOATING - Do not scroll the object when the parent scrolls and ignore layout
-
FLAG_OVERFLOW_VISIBLE - Do not clip the children’s content to the parent’s boundary
3. BunTalk script
3.1. BunTalk syntax
BunTalk is a simple script language. The statement must be end with semicolon ";". Like following code.
ptr("hello world"); // print "hello world" to UART
label.text("Welcome"); // Set label text to "Welcome"
3.2. Keywords
BunTalk has following keywords
if, else, and, or
these keywords can’t be used as object(widget/screen) name.
3.3. Arithmetic and bitwise operation
BunTalk support basic arithmetic [ + , - , * , / , %] and () operations
BunTalk support bitwise [ &, |, ^] operations
ptr(5+6); // print 11 to UART
ptr((5+9)%10); // print 4 to UART
ptr(7&3); // print 3 to UART
ptr(5|2); // print 7 to UART
ptr(5^1); // print 4 to UART
label.text(bar.val()*2); // Set label text to value of bar multiply 2
label2.text((bar.val() + bar2.val()) / 2); // Set label2 text to (bar.val()+bar2.val()) / 2
3.4. Flow control and logical operation
BunTalk support flow control and logical operation
Syntax |
operation |
! |
Logical inverse |
== |
Equal |
!= |
Not equal |
> |
Great then |
>= |
Great or equal then |
< |
Less then |
< = |
Less or equal then |
and |
logical AND |
or |
logical OR |
Example code of flow control and logical opertaion
// if statement
if(sw.checked())
{
ptr("checked");
}
// if-else statement
if(bar.val() > 50)
{
label.hidden(0); // Show label
label.text(bar.val()); // Set label text
}else{
label.hidden(1); // hide label
}
// logical AND and great then operation
if(sw.checked() and (slider.val() > 50))
{
ptr("slider=", slider.val());
}
// logical OR operation
if(sw.checked() or sw2.checked())
{
led.color(rgb16(255, 0, 0));
}else{
led.color(rgb16(0, 255, 0));
}
3.5. BunTalk Datatype
There 3 major data type
-
Number: Integer number.
-
String: String is surround by double quote and is encoded with UTF-8 format.
-
Object: Most objects are widget object, except sys and screen object. Objects are created in BunMaker.
3.6. BunTalk by UART
You can send BunTalk by UART to interact with BunHMI. When host transmit BunTalk by UART, you must add <EOT>(04h) at the end of command string. (<EOT> stand for END OF Transmitssion). And the max BunTalk script length on BunHMI UART buffer is 256 bytes.
When using arduino, you can use Serial.print to send BunTalk to BunHMI. Here is an arduino example
// Method 1:
#define EOT 0x04
char buff[256];
snprintf(buff, sizeof(buff), "ptr(\"Hello\"); bar.val(%d);", val);
Serial1.print(buff);
Serial1.print("lab.text(\"Some Text\");"); // Send more script
Serial1.write(EOT); // Then send EOT at the end.
// Method 2:
snprintf(buff, sizeof(buff), "widget.text(\"CNT:%d\");\x04", test_cnt++); // add \x04 at the end of script
Serial.print(buff);
You can choose either Method 1 or Method 2 which is appropriate.
The BunTalk script widget.text() is to invoke widget’s method, We will talk about widgets and methods at Section 7 later.
When you want receive data from BunHMI, here is the example code of arduino
#define EOT 0x04
// the setup routine runs once when you press reset:
void setup() {
// initialize serial communication at 115200 bits per second:
Serial1.begin(115200);
// Set UART Rx Time out to 10ms
Serial1.setTimeout(10);
}
// Check and receive data from BunHMI
int rxBunHmiData(char* buff, int buff_len) {
static char hmiBuff[256];
static uint8_t hmiBuffLen = 0;
if (!Serial1.available()) {
return 0;
}
int rxlen = Serial1.readBytes(hmiBuff + hmiBuffLen, sizeof(hmiBuff) - hmiBuffLen);
int i;
hmiBuffLen += rxlen;
// Search EOT token from the end of hmiBuff
for (i = hmiBuffLen - 1; i >= 0; i--) {
if (hmiBuff[i] == EOT) {
// Got EOT Byte
hmiBuff[i] = 0; // Replace EOT to NULL (string terminate)
i++;
int hmi_len = (i < buff_len) ? i : buff_len;
// Copy hmiBuff to buff
memcpy(buff, hmiBuff, hmi_len);
// Move remain data to the head of hmiBuff
int remain_len = 0;
while (i < hmiBuffLen) {
hmiBuff[remain_len++] = hmiBuff[i++];
}
hmiBuffLen = remain_len;
return hmi_len;
}
}
return 0;
}
// the loop routine runs over and over again forever:
void loop() {
char rxbuff[256];
int rxlen;
// Check Data from HMI
rxlen = rxBunHmiData(rxbuff, sizeof(rxbuff));
if (rxlen) {
// Handle data from HMI
// TODO: add handleHmiData(rxbuff);
}
}
For easy understanding, the following document won’t show <EOT> explicitly.
3.7. BunTalk in BunMaker
You can use BunTalk script in BunMaker too. When use BunTalk in BunMaker, there is no need <EOT> at the end of BunTalk script command.
In BunMaker, there is an Events Panel (Figure 7) in widget settings. You can write BunTalk script in events panel.
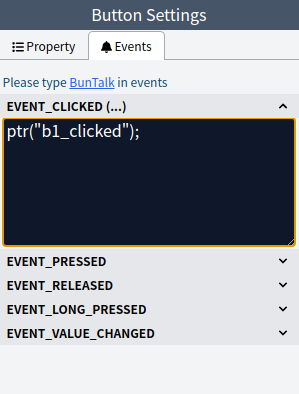
Above settings will print b1_clicked to UART to notify host that Button is clicked.
You can add comments in Events Panel as well. Comments are start with "//" to the end of line, like following figure.
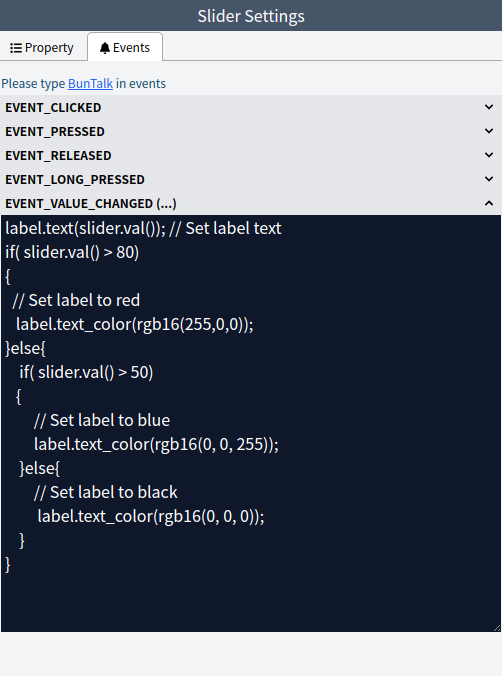
3.8. Error message
When BunTalk script arise some error, It will spit out error message with prefix: !!.
For example, if you send wrong BunTalk message to BunHMI, it will send error message:
-
Run BunTalk: ptr("hello"
-
Get Response: !!Error at end: Expect ')' after arguments.
Also, if BunTalk script in BunMaker arise runtime error, it will spit out error message to UART.
3.9. Event message
When running animation, we can set event trigger when animation is done. BunHMI will send event message with prefix: !^.
For example
-
!^EVT_ANIM_X_END:widget_name
-
!^EVT_ANIM_VAL_END:widget_name
-
!^WAV_PLAY_END
3.10. Color format
BunHMI use RGB565 16bit color format
. That is use a 16bit binary code to represent RGB color channel.
-
Red: 5bits, bit[15..11]
-
Green: 6bits, bit[10..5]
-
Blue: 5bits, bit[4..0]
A number will translate as a 16bit binary code to represent a RGB565 color code.
4. Global Functions
The following table show globol funtion in BunTalk
Function | arguments & return | Desc. |
---|---|---|
atoi(str) |
args: |
atoi() is similar to atoi() function of standard C function. ex:
|
ptr(…) |
args: <Number|String>, ptr() accept variable arguments |
ptr() is used to send string to BunHMI UART Tx let host make response by the message. ex: print widget width NOTE: ptr() will add EOT(04h) at the end of string. |
strcat(…) |
args: <Number|String>, strcat() accept variable arguments ex: Set widget text If value of bar is 30, this will set widget’s text to display Note: widget.text() & bar.val() are widget methods that described in Section 7. |
strcat() is used to concatenate args to string. It is used to set widget text mostly. |
rgb16(r,g,b) |
args: ex: Set widget color to red return: Number: Represent RGB565 color format |
This function is used to convert red,green,blue color channel to RGB565 color format |
5. Sys Methods
Sys is a globol object. you can access Sys by name sys. Like this code
sys.beep(2000, 100); // Trigger BunHMI onboard beeper.
sys.bright(60); // Set LCD backlight to 60%
sys.scr(scr2); // Change screen to scr2
5.1. Variables
The sys object includes 16(0~15) variables for save temporary data. The value can be a number, string or object. These values are stored at RAM and will reset after power cycle.
// To write variable
sys.var(0, 11.2); // assign number to variable, var[0] = 11.2
sys.var(1, "test"); // assign string to variable, var[1] = "test"
sys.var(2, label); // assign object to variable, var[2] = widget
// To read variable
ptr(sys.var(0)); // print 11.2
ptr(sys.var(1)); // print "test"
sys.var(2).text("Hello"); // Set label text to "Hello"
5.2. load_str(), save_str()
Use load_str(), save_str(), To save string data in nonvolatile flash memory. The max length of one slot is 256 bytes.
This can be useful to save product information in BunHMI or save some nonvolatile data.
sys.save_str(10, "SN:SKU123465"); // save string at offset 10 bytes of flash memory;
ptr(sys.load_str(10, 12)); // print string from flash memory with offset 10 bytes and 12 bytes length.
5.3. Message box
To display message box, you can call sys.mb_show() like this
sys.mb_show("Test", "This is test mesg", "OK", "Cancel"); // Display message box
Display message box like this
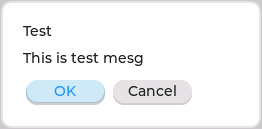
BunHMI will send string OK to UART when user click OK button and send string Cancel to UART when user click Cancel button.
5.4. Gesture Event
You can get gesture direction by invoke sys.gesture() method in EVENT_GESTURE.
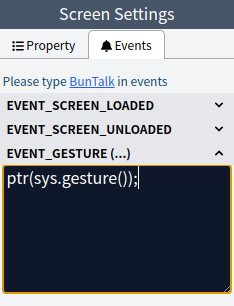
Most widget has default flag: FLAG_GESTURE_BUBBLE enabled. which will pass gesture event to its parent and will not receice EVENT_GESTURE.
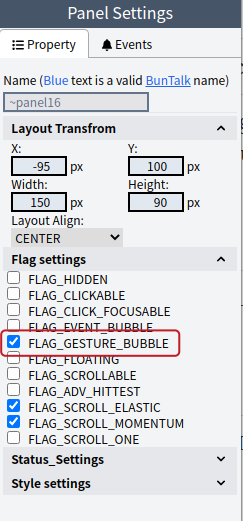
If you need get gestures on a widget, please disable FLAG_GESTURE_BUBBLE flag of the widget to get the EVENT_GESTURE.
If all widgets pass gesture event to its parent, then the Screen will get gesture event at finally.
5.5. System tick
System tick can be used to run script periodically. You can find system tick settings in the Project setting of Toolbox.
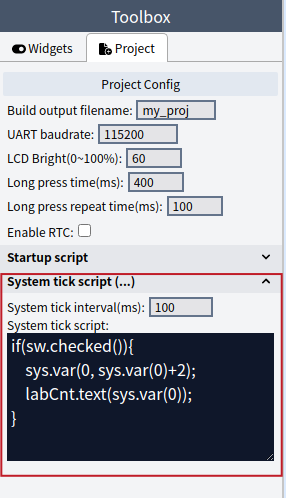
System tick interval: this value define tick interval in mini second(ms), that System tick script will be run for every tick interval.
Caution
|
When using system ticks, you must pay attention to whether the widget name exist in current display screen. That’s say if there is a widget named:"lab1" which is only exist in Screen1, and used by System ticks script. When HMI change to Screen2 the "lab1" is no longer accessable, which will cause error!! |
We suggest using Screen Tick, if you need control widgets for each screen.
5.6. Timer
Timer just like system tick, which can run BunTalk script periodically. and you can change the running scirpt in runtime by using sys.timer().
sys.timer(0, 0, 500, 1, "if(sw.checked()){label.text(sys.rtc_str(0));}");
This will set timer to run following code for every 500ms
if(sw.checked()){label.text(sys.rtc_str(0));}
// update label text to RTC time for every 500ms if sw widget is checked
If you want to cancel or stop timer, you can using following method, just pass timer id without any other arguments
sys.timer(0); // Stop timer0
sys.timer(2); // Stop timer2
5.7. Methods list
Method |
arguments & return |
Desc. |
beep(hz, ms) |
args: return: none |
Trigger BunHMI on board beeper, to generate frequency for millisecond. |
bright(val) |
args: return: none |
Set LCD backlight |
bright() |
args: none return: <Number>, LCD backlight bright |
Get LCD backlight |
gesture() |
args: none return: <Number>, gesture dir bitmap
|
Get gestures dir. This is ofen called in EVENT_GESTURE ![]() |
load_str(pos, len) |
args: return: <String>, String read from flash memory. |
Load string from BunHMI non-volatile flash memory. BunHMI proide 256bytes non-volatile memory. To load string from non-volatile memory, you can use load_str() like Read load string
This will print string from non-volatile memory at position 10. with max string length is 50 |
map(value, fromLow, fromHigh, toLow, toHigh) |
args: return: <Number>, The mapped value. |
Re-maps a number from one range to another. Note that the "lower bounds" of either range may be larger or smaller than the "upper bounds" so the map() function may be used to reverse a range of numbers, for example y = map(x, 1, 50, 50, 1); The function also handles negative numbers well, so that this example y = map(x, 1, 50, 50, -100); is also valid and works well. |
mb_show(title, mesg, btn1, btn2, …) |
args: return: none |
Show message box
This will display message box with 2 buttons. You can use 1, 2 or 3 buttons, depend on how many arguments you passed. If button is clicked, BunHMI will send button text to UART. ie: if Button OK is clicked, you will get "OK" by UART. And message will disappear, if any button is clicked. |
mb_close() |
args: none return: none |
Close message box |
rand(max) |
args: return: <Number>, Random number |
Get random number in range between min~max If only max is provided, the range will be 0~max |
scr(screen) |
args: return: none |
Load screen |
scr(screen, anim_type, time, delay) |
args: anim_type <Number>:
time: <Number>, time of the animation, in millisecond delay: <Number>, delay before the transition, in millisecond return: none |
Load screen with animation |
scr() |
args: none return: <Object>, The current display screen object |
Get current display screen object, please refer [Screen] for more screen methods |
stop_anim() |
args: none return: none |
Stop all animations. |
save_str(pos, string) |
args: return: none |
Save string to BunHMI non-volatile flash memory. BunHMI proide 256bytes non-volatile memory. You can use these memory to save some product information like Serial Number or Version Number. To save string to non-volatile flash memory, you can use save_str() like
This will save string "12345" to flash memory at offset position 10. |
timer(id, delay, interval, repeat, script) |
args: return: none |
Set timer to run BunTalk script. There are 8 timers you can use. Use id to select which timer you want to use, and set the delay, interval and repeat to run BunTalk script. timer example
This will set timer0 to run script led.toggle() repeatedly wieh interval 100ms, after 500ms delay. Note: When new screen is loaded, old screen timer will be all stopped. |
timer(id) |
args: return: none |
Stop timer. Use this method to stop timer. |
touch_en(enable) |
args: enable, <Number> return: None |
Enable/Disable touch screen function. |
var(id) |
args: return: <Number|String|Object> |
Read variable slot. |
var(id, val) |
args: return: none |
Save val to variable slot. |
6. Screen
Screen object can be got by sys.scr(), which is return current display screen object.
Examples of how to use Screen object.
ptr(sys.scr().name()); // Print current screen name
sys.scr().bg_img(1); // Set screen background to image id 1
sys.scr().bg_color(rgb16(255,0,0)); // Set screen background to red
6.1. Screen tick
Screen tick can be used to run BunTalk script periodically. The script will only be run when screen is displayed. You can find screen tick settings in the Screen settings panel.
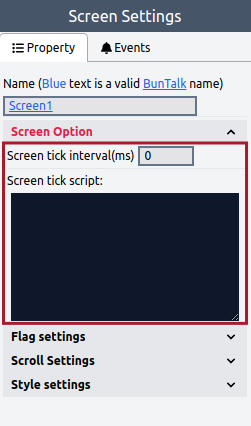
Screen tick interval: this value define tick interval in mini second(ms), that Screen tick script will be run for every tick interval.
6.2. Access unloaded screen widgets
For widgets in current display screen, you can direct access widget by widget name.
However, if screen is not loaded on display, you have to access widget by screen property, like
label1.text("Hello"); // For current display screen, you can access widget by widget name directly.
Screen1.label1.text("Hello"); // or access widget by screen property, nomatter screen is display or not
When you call sys.scr(); to load new screen, widgets that is not in new screen can only be accessed by screen property.
This is particularly used in screen unloaded event, if you need access the widget when screen is unloaded. You can use screen property to access the widget for unloaded screen.
For example, you have a Screen1 with label1 widget, you can use label1.text() at EVENT_SCREEN_LOADED because Screen1 is loaded, but you have to use Screen1.label1.text() at EVENT_SCREEN_UNLOADED to access the label1 due to Screen1 is not loaded, like Figure 12.
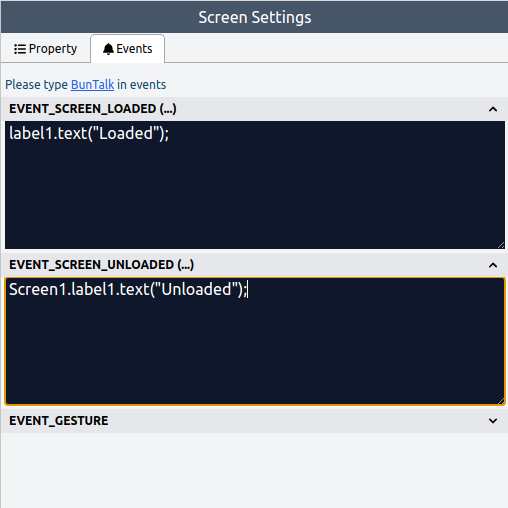
Method |
arguments & return |
Desc. |
name() |
args: return: <String>, Screen name |
Get name of screen |
bg_img(var) |
args: return: none |
If var is number. This will set image source by image id. Image ID is assigned in BunMaker editor. If var is string. This will set image source by filename in SDCard, the format of image may be jpg, png or bmp file. |
bg_color(var) |
args: return: none. |
Set background color |
font(name) |
args: name: <String>, embed font name return: none |
Set the embed font of screen. The embed fonts includes all buildin fonts
plus all embed fonts that add by user. Ex: set embed font of screen
|
7. Widgets
7.1. Widget Base
Widget is created by Bunmaker, you can call widget method by widget name which is set in BunMaker.
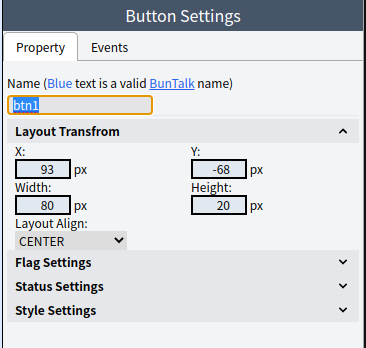
Like btn1.width(), the btn1 is widget name, the width() method will return the width of the widget.
Method |
arguments & return |
Desc. |
bg_color(color) |
args: return: none. |
Set background color of widget Ex: Set background color to RED
|
bg_grad_color(color) |
args: return: none. |
Set background gradient color of widget |
bg_grad_stop(main, gard) |
args: return: none. |
Set the stop of main color and start of gradient color in percentage(%). |
bg_grad_dir(dir) |
args: |
Set direction of gradient color. |
bg_opa(val) |
args: return: none. |
Set background opacity of the widget |
clickable(val) |
args: return: none. |
Set widget clickable flag. |
clickable() |
args: none return: <Number>, the clickable flag. |
Get the clickable flag of the widget. |
co_x() |
args: none return: <Number>, the x position relative to screen. |
Get the x position relative to screen. |
co_y() |
args: none return: <Number>, the y position relative to screen. |
Get the y position relative to screen. |
disabled(val) |
args: return: none. |
To enable/disabled widget. |
disabled() |
args: none return: <Number>, the disabled flag. |
Get the disable flag of the widget. |
focused(val) |
args: return: none. |
To set focused of widget. |
focused() |
args: none return: <Number>, the focused flag. |
Get the focused flag of the widget. |
font(name) |
args: name: <String>, embed font name return: none |
Set the embed font of widget. The embed fonts includes buildin fonts
plus all embed fonts add by user. Ex: set embed font of widget
|
height() |
args: none return: <Number>, the height of widget. |
Get the width of widget. |
height(val) |
args: return: none. |
Set the height of widget. |
hidden(val) |
args: return: none. |
To show/hide widget. |
hidden() |
args: none return: <Number>, the hidden flag. |
Get the hidden flag of the widget. |
opa(val) |
args: return: none. |
To set opcity of widget |
opa() |
args: none return: <Number>, the opacity value. |
Get the opacity value of widget. |
pos(x,y) |
args: return: none |
Set the transformed position x,y value of widget. |
size(w,h) |
args: return: none |
Set the size of widget. |
text_color(val) |
args: return: none. |
Set text color of the widget and widget’s children |
text_opa(val) |
args: return: none. |
Set text opacity of the widget and widget’s children |
width() |
args: none return: <Number>, the width of widget. |
Get the width of widget. |
width(val) |
args: return: none. |
Set the width of widget. |
x(val) |
args: return: none |
Set the transformed position x value of widget. |
x() |
args: none return: <Number>, the transformed position x of widget. |
Get the transformed position x value of widget relative to parent widget. |
y(val) |
args: return: none |
Set the transformed position y value of widget. |
y() |
args: none return: <Number>, the transformed position y of widget. |
Get the transformed position y value of widget relative to parent widget. |
7.1.1. Animation Methods
The animation methods get a little complicated. But they are quite similar.
This table show how to animation on x properties of widget, other properties are apply in similar way.
If you want stop all animations, you can use
sys.stop_anim()
This will stop all animations.
And when a new screen is loaded, all animations in old screen will be stoped.
7.2. Arc
Arc widget inherit all methods from Table 6. Plus following methods
Method |
arguments & return |
Desc. |
val(val) |
args: return: none |
Set value |
val() |
args: none return: <Number>, value of arc |
Get arc value |
angle(start, end) |
args: return: none |
Set arc angle. Zero degrees is at the middle right (3 o’clock) of the object and the degrees are increasing in clockwise direction. |
angle() |
args: none return: <String>, start,end value of angle |
Get arc angle, if start angle is 120, stop angle is 60 |
bg_angle(start, end) |
args: return: none |
Same as angle(start, end), this set the background angle of arc. |
rotation(val) |
args: return: none |
Set arc rotation angle |
range(min, max) |
args: return: none |
Set value range. |
range() |
args: none return: <String>, min,max |
Get range, if range min is 0, range max 100 |
anim_val |
refer: |
Animatie widget property |
7.3. Bar
Bar widget inherit all methods from Table 6. Plus following methods
Method |
arguments & return |
Desc. |
val(val,[anim=0]) |
args: anim: <Number>, 0: No animation, 1: With animation return: none |
Set value |
val() |
args: none return: <Number>, bar value |
Get value |
start_val(val) |
args: return: none |
Set start value |
start_val() |
args: none return: <Number>, start value |
Get start value |
range(min, max) |
args: return: none |
Set value range. |
range() |
args: none return: String, min,max |
Get range, if range min is 0, range max 100 |
anim_val |
refer: |
Animatie widget property |
7.4. Button
Button widget inherit all methods from Table 6.
7.5. Calendar
Calendar widget inherit all methods from Table 6. Plus calendar methods
7.5.1. Get user pressed date
You can get user pressed date by add BunTalk script to EVENT_VALUE_CHANGED like
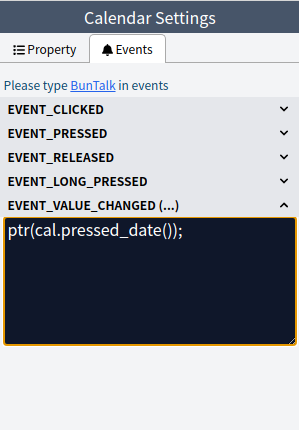
When user press the date on calendar, BunHMI will print the date string that user presssed like: 2020,12,1
Method |
arguments & return |
Desc. |
today_date(year,month,day) |
args: return: none |
Set today date |
today_date() |
args: none return: <String>, today date. |
Get today date. if today is 2020/1/1, this will return 2020,1,1 |
showed_date(year,month) |
args: return: none |
Set showed date |
showed_date() |
args: none return: <String>, The year and month of showed date. |
Get year and month of showing date. if showed date is 2020/1, this will return 2020,1 |
pressed_date() |
args: none return: <String>, get user pressed date. |
Get user pressed date. if presse date is 2020/1/1, this will return 2020,1,1 |
7.6. Chart
Chart widget inherit all methods from Table 6. Plus chart methods.
7.6.1. Chart type
Chart has following types.
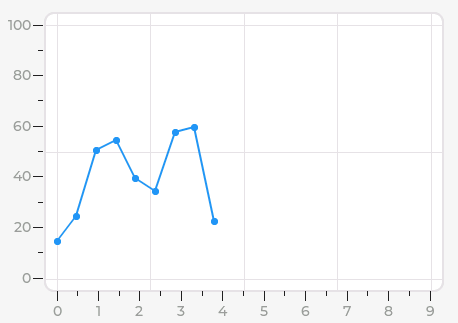
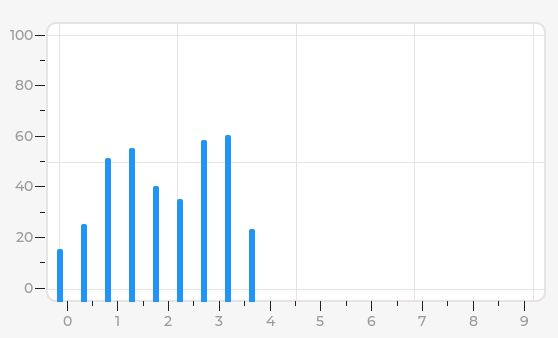
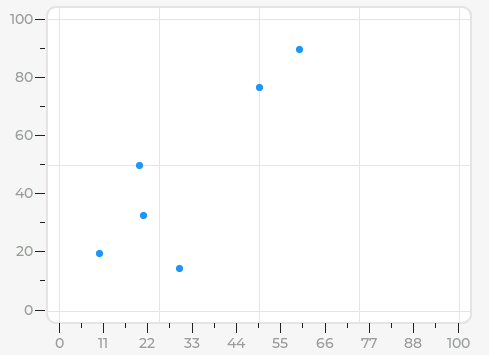
7.6.2. Custom X Label
For line chart or bar chart, the default x label is <Number> sequence start from 0. X label can be changed by in chart option
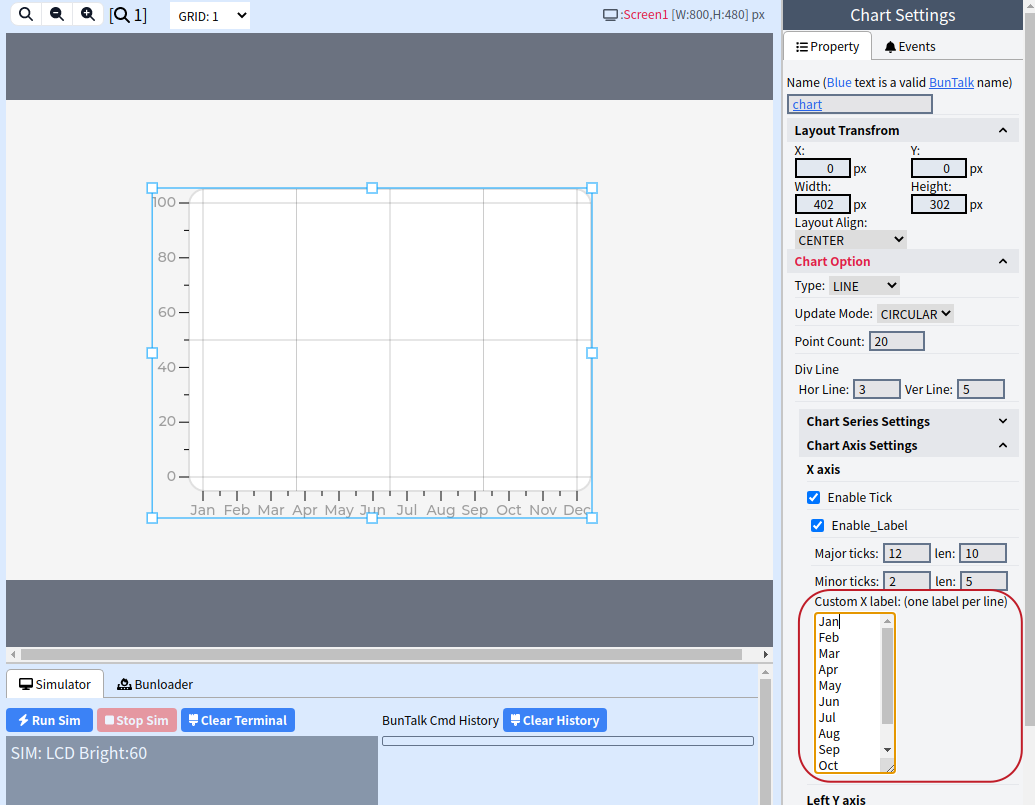
Edit Custom X label(one label per line) in X axis settings.
7.6.3. Line chart style
The default line chart is look like as below
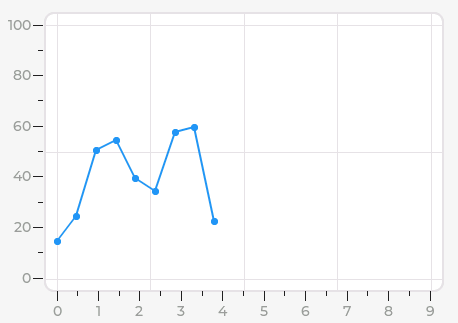
You can set Paint size of PART_INDICATOR to 0, to hide the dot.
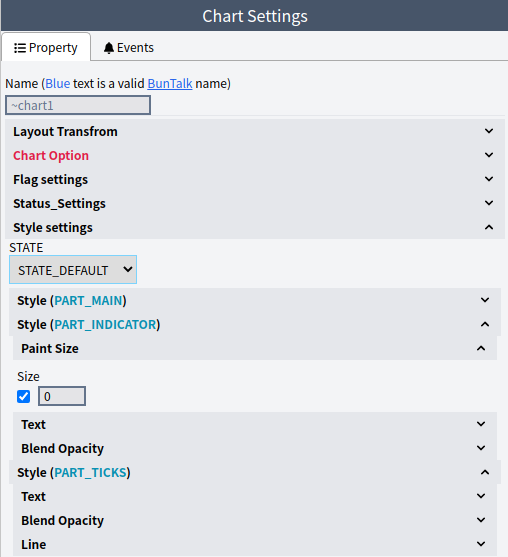
The line chart without dot.
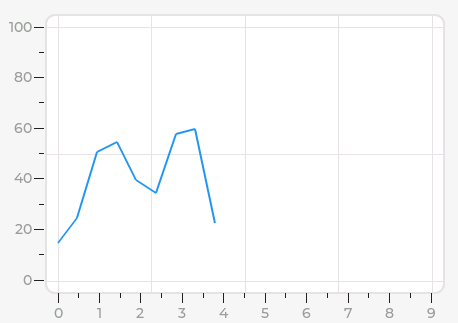
7.6.4. How to modify chart data
The data in chart are represent by series. The first series id is start by 0.
You have several options to set the data of series:
Set next data
Set the next data of series and refresh chart
chart.next(0, 100); // set next value of series0 to 100
chart.mnext(30, 20); // set next value of series0 to 30 and series1 to 20
chart.next2(0, 10, 20);// In scatter chart, you can set (x,y) value for each data
Set data value
Set data value by id or for all data and refresh chart
chart.set_value(0, 10, 33); // Set series0[10]=33 and refresh chart
chart.set_all(0, 100); // Set all data of series0 to 100
Set data array of series
If you need display chart fast. you can set multiple data and refresh the chart at last. Which can update multiple data at once.
chart.ser_i(0, 10); // select series 0, set update index to 10
chart.ser(10,11,12,13,14,15,16,17,18,19); // update values, max argument count is 10
chart.ser(20,21,22,23,24,25); // update more values
chart.refresh(); // refresh chart.
This is quite useful when you want display a completed chart at screen load.
7.6.5. Reset chart data
There is a special value: 32767, which is stand for non point. The chart widget will skip this value and not draw it.
If you want to clear or reset a series data, you can wrote
chart.set_all(0, 32767); // Clear data of series0
Method |
arguments & return |
Desc. |
div_line(hdiv, vdiv) |
args: return: none |
Set number of div line |
get_point_pos(ser, id) |
args: return: String |
Get point position, if point is at x:10, y:30, this method will return 10,30 |
mnext(…) |
args: <Number>, multiple arguments return: none |
Set next value to multiple series ex: widget.mnext(10,22,15) |
next(ser,val) |
args: return: none |
Set next value of series |
next2(ser, x_val, y_val) |
args: return: none |
Set next x,y values of series. This is only for scatter chart. |
point_count(val) |
args: return: none |
Set point count of chart. |
point_count() |
args: none return: <Number>, pointer count |
Get point count of chart |
refresh() |
args: none return: none |
Force refresh the chart. you need call chart.refresh() after call chart.ser() to force chart update. |
ser(val1, val2, …) |
args: return: none |
Add value to series data at begin of index that set by method ser_i().
The data index will auto increment and back to 0 after reach the end of data. You can call chart.ser() multiple times to update all the series data, then call chart.refresh() to update the chart. |
ser_color(ser, color) |
args: return: none |
Set series color |
ser_hide(ser, hide) |
args: return: none |
Set show/hide series |
ser_i(ser, idx) |
args: return: none |
Set data update index of series, this will take effect on chart.ser() method. |
ser2(x1,y1,x2,y2 …) |
args: return: none |
This method just like ser(), but this is only for scatter chart which need (x,y) value for every data point.
The data index will auto increment and back to 0 after reach the end of data. You can call chart.ser2() multiple times to update all the series data, then call chart.refresh() to update the chart. |
set_all(ser, val) |
args: return: none |
Set all data set of series |
set_value(ser, id, val) |
args: return: none |
Set particular data point of series |
set_value2(ser, id, x_val, y_val) |
args: return: none |
Set particular data point of series. This is only for scatter chart. |
set_range(ser, min, max) |
args: return: none |
Set range of series |
type(type) |
args: return: none |
Set chart type |
update_mode(mode) |
args: return: none |
Set update mode |
x_start(ser, point) |
args: return: none |
Set x start point |
x_start(ser) |
args: return: <Number> |
Get x start point of series |
zoom_x(val) |
args: return: none |
Set zoom factor of x axis, 128=50%, 256=100%, 512=200% and so on. |
zoom_x() |
args: none return: <Number>, zoom factor |
Get zoom factor of x axis |
zoom_y(val) |
args: return: none |
Set zoom factor of y axis, 128=50%, 256=100%, 512=200% and so on. |
zoom_y() |
args: none return: <Number>, zoom factor |
Get zoom factor of y axis |
7.7. Checkbox
Checkbox widget inherit all methods from Table 6. Plus following methods
Method |
arguments & return |
Desc. |
text(val) |
args: return: none |
Set display text |
text() |
args: none return: <String>, text. |
Get display text. |
checked(val) |
args: return: none |
Set checkbox check status |
checked() |
args: none return: <Number>, 0: checked, 1: unchecked |
Get checkbox check status |
7.8. Drop-down
Drop-down menu is a commonly used UI element.
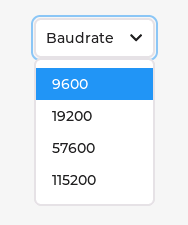
Here we name a drop-down menu as dd, and set drop-down options with "9600\n19200\n57600\n115200". So user can choose one of these options.
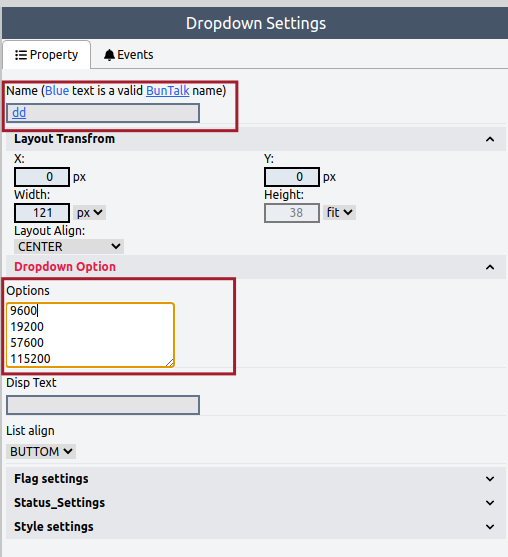
In the events settings panel of drop-down widget, wrote BunTalk script in the EVENT_VALUE_CHANGED event.
ptr("You SEL:", dd.selected_str()); // Print selected item string
dd.text(dd.selected_str()); // Set dropdown to display selected item
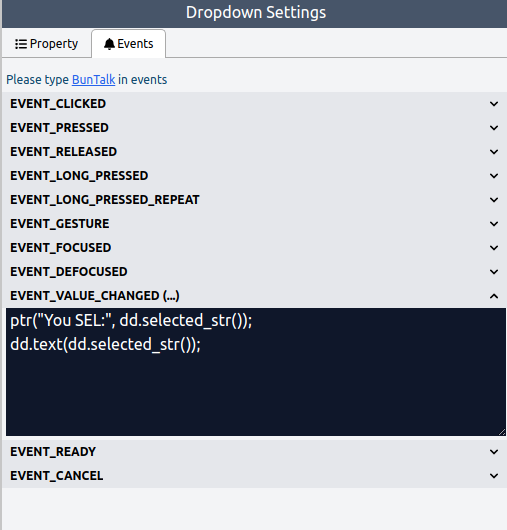
Drop-down widget inherit all methods from Table 6. Plus following methods
Method |
arguments & return |
Desc. |
options(str) |
args: return: none |
Set dorp-down menu options.
|
options() |
args: none return: <String>, options string. |
Get dorp-down menu options string. |
add_option(str, pos) |
args: return: none. |
Append option item to dorp-down menu. |
clear_options() |
args: none return: none. |
Clear all dorp-down menu options |
selected(pos) |
args: pos: <Number>, select position return: none. |
Set dorp-down menu select position |
selected() |
args: none return: <Number>, selected position. |
Get selected position of dorp-down menu |
selected_str() |
args: none return: <String>, selected item string. |
Get selected item string. |
text(val) |
args: return: none |
Set display text or <Number> of dorp-down menu |
text() |
args: none return: <String>, title text. |
Get display text of dorp-down menu. |
dir(val) |
args: return: none |
Set popup menu display direction |
dir() |
args: none return: <Number>, disp dir, refer dir(val) method. |
Get popup menu display dir |
highlight(val) |
args: <Number>, 0: disable high-light, 1: enable high-light return: none |
Set high-light status |
highlight() |
args: none return: <Number>, 0: high-light is disable, 1: high-light is enable |
Get high-light status |
open() |
args: none return: none. |
Open dorp-down menu |
close() |
args: none return: none. |
Close dorp-down menu |
is_open() |
args: none return: <Number>. 0: menu is close, 1: menu is open |
Get dorp-down menu open status. |
option_cnt() |
args: none return: <Number>, number of options. |
Get dorp-down options count. |
7.9. Image
Image widget inherit all methods from Table 6. Plus image methods
7.9.1. Rotation
Rotate the image by angle() method. Set appropriate pivot() before rotate the image. Check following image rotation with different pivot
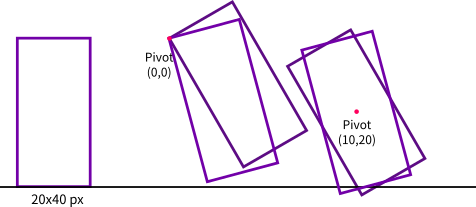
7.9.2. Recolor
A color can be mixed with every pixel of an image with a given intensity. This can be useful to show different states (checked, inactive, pressed, etc.) of an image without storing more versions of the same image.
You can use recolor() to set the color and enable this feature by recolor_opa() between (no recolor, value:0) and (full recolor, value:255). The default value is 0, so this feature is disabled.
For example, the following image
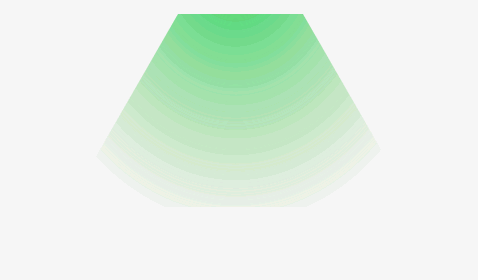
You can change the image color by using
image.recolor(rgb16(200,0,0));
image.recolor_opa(200);
This turns out
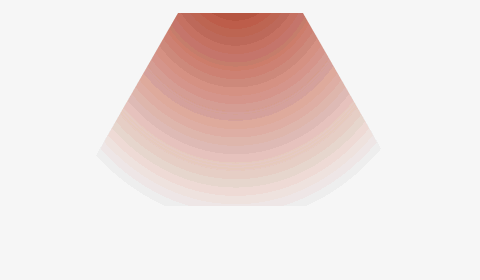
Method |
arguments & return |
Desc. |
angle(val) |
args: return: none |
Set rotation angle |
angle() |
args: none return: <Number>, Rotation angle. |
Get rotation angle. |
zoom(val) |
args: return: none |
Set zoom factor, 128=50%, 256=100%, 512=200% and so on. |
zoom() |
args: none return: <Number>, zoom factor |
Get zoom factor. |
src(var) |
args: return: none |
If var is number. This will set image source by image id. Image ID is assigned in BunMaker editor. If var is string. This will set image source by filename in SDCard, image format may be jpg, png or bmp file. Note: If you select image source in Bunmaker is not a gif image, Don’t use src() to assign a gif image. It won’t showup. |
offset_x(val) |
args: val: <Number>, offset x return: None |
Set image display offset x value. |
offset_x() |
args: none return: <Number>, offset x |
Get image display offset x value. |
offset_y(val) |
args: val: <Number>, offset y return: None |
Set image display offset y value. |
offset_y() |
args: none return: <Number>, offset y |
Get image display offset y value. |
pivot(x, y) |
args: return: None |
Set image rotation pivot. |
pivot() |
args: None return: <String>, pivot value with the format "x,y" |
Get image rotation pivot. |
recolor(color) |
args: return: none. |
Set image recolor |
recolor_opa(val) |
args: return: none. |
Set image recolor opacity. |
anim_angle |
refer: |
Animatie widget property |
7.10. ImgBtn
Imgbtn widget inherit methods all from Table 6.
7.11. Keyboard
Keyboard widget has 2 special key that will trigger event.
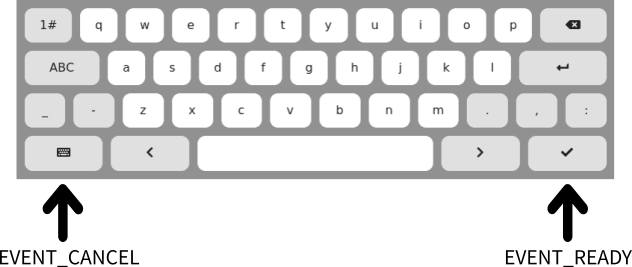
You can write BunTalk in event handler when these key is pressed.
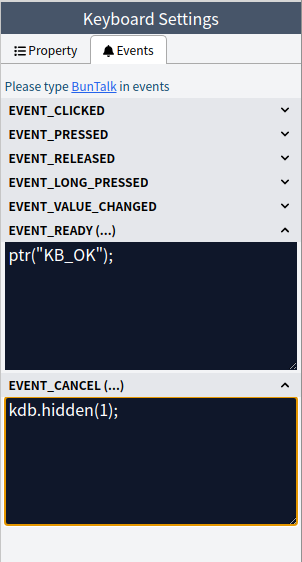
Keyboard widget inherit all methods from Table 6. Plus following methods
Method |
arguments & return |
Desc. |
mode(val) |
args: return: none |
Set keyboard mode |
mode() |
args: none return: <Number>. refer val list in mode() method. |
Get kayboard mode. |
popover(val) |
args: <Number>: 0 disable popover, 1: enable popover return: none. |
Set show the button title in a popover when pressed. |
popover() |
args: none |
Get popover status. |
textarea(obj) |
args: <Object>: widget name of Textarea return: none |
Set keyboard target textarea widget |
ptrkey(prefix, [ctrl_only=0]) |
args: ctrl_only: <Number>, 0: print all key char, 1: print control key only return: None |
Print the last key of keyboard pressed. This will be used in EVENT_PRESSED of keyboard widget usually. Normal key are like 0-9,A-Z,…; Where control key are like backspace, left, right … Control key are listed below, the print char which will contains more than one char When pass and set ctrl_only=1, this will print control key only.
|
7.12. Label
Label widget inherit all methods from Table 6. Plus following methods
Method |
arguments & return |
Desc. |
text(val) |
args: return: none |
Set label display text or number |
text() |
args: none return: <String>, Label text. |
Get label display text. |
7.13. Led
Led widget inherit all methods from Table 6. Plus following methods
Method |
arguments & return |
Desc. |
on(val) |
args: return: none |
Set Led on/off |
toggle() |
args: none return: none |
Set Led state between on/off |
bright(val) |
args: return: none |
Set Led brightness |
bright() |
args: none return: <Number>, Led brightness(0~255) |
Get Led brightness |
color(color) |
args: return: none |
Set Led color |
anim_bright |
refer: |
Animatie led bright |
7.14. Line
Line widget inherit all methods from Table 6. Plus following methods
Method |
arguments & return |
Desc. |
color(color) |
args: return: none |
Set Line color |
mov_p(idx, x, y) |
args: return: none |
Move coordinate of on point and update line drawing.
|
set_p(idx, x, y) |
args: return: none |
Set coordinate of one point. but not update line drawing. To update line drawing call refresh() to refresh line drawing.
|
refresh() |
args: none return: none |
Refresh line drawing |
7.15. Panel
Panel widget inherit all methods from Table 6.
7.16. QRCode
QRCode widget inherit all methods from Table 6. Plus following methods
Method |
arguments & return |
Desc. |
update(str) |
args: return: none |
Update qrcode to show new string. |
7.17. Roller
Roller widget inherit all methods from Table 6. Plus following methods
Method | arguments & return | Desc. |
---|---|---|
options(str, [mode=0]) |
args: return: none. |
Set roller options
|
options() |
args: none return: <String>, options string. |
Get options string. |
selected(pos, [anim=0]) |
args: return: none. |
Set select position |
selected() |
args: none return: <Number>, selected position. |
Get selected position |
selected_str() |
args: none return: <String>, selected item string. |
Get selected item string. |
7.18. Slider
Slider widget inherit all methods from Table 6. Plus following methods
Method |
arguments & return |
Desc. |
val(val,[anim=0]) |
args: return: none |
Set value |
val() |
args: none return: <Number>, value of slider |
Get value |
left_val(val,[anim=0]) |
args: return: none |
Set left value |
left_val() |
args: none return: <Number>, left value of slider |
Get left value |
range(min, max) |
args: return: none |
Set value range. |
range() |
args: none return: <String>, min,max |
Get range, if range min is 0, range max 100 |
anim_val |
refer: |
Animatie widget property |
7.19. Switch
Switch widget inherit all methods from Table 6. Plus following methods
Method |
arguments & return |
Desc. |
checked(val) |
args: return: none |
Set switch check status |
checked() |
args: none return: <Number>, 0: checked, 1: unchecked |
Get switch check status |
7.20. Textarea
Textarea widget inherit all methods from Table 6. Plus following methods
Method |
arguments & return |
Desc. |
text(val) |
args: return: none |
Set text of Textarea |
text() |
args: none return: <String>, text. |
Get text of Textarea |
add_text(var) |
args: return: none |
Add text, you can add string or number. |
del_char(forward) |
args: return: none |
Del char in textarea. |
cursor_pos(pos) |
args: return: none |
Set cursor pos |
cursor_move(dir) |
args: return: none |
Move cursor dir |
8. SD Card
There is one SD card slot on BumHMI, you can access SD Card by Buntalk. BunHMI leverage LVGL filesystem library to access SD Card. Most API are similar with LVGL filesystem.
8.1. File descriptor
Due to resource constraints BunHMI only allow limited file descripts. Currently, you can open 4 files at the same time. If you need access another file, the original opened file will be closed.
8.2. API in sys object
SD Card API is included in sys object. So you can invoke file system API like
/* print directory content */
ptr(sys.f_dir(""));
/* open file */
sys.f_open(0,"/test2.txt", 1);
This will open SD Card file "/test2.txt" in write mode, and use file descriptor 0.
Method |
arguments & return |
Desc. |
f_dir(path, [offset=0], [cnt=0]) |
args: If only path is passed, the offset and cnt is set to 0 return: <String>, directory list, The max length of string is 512 |
This will list directory content. like /image\n The string start with / is a directory. The max return string length is 256Bytes, if the returned directory’s content exceed 256Bytes, it will show '…' at the end like /image\n You can use offset to skip files items, that will not includes in return string. You can use cnt to limit files list count, that only includes cnt number of files in return string. if cnt=0, there will be no count limitation. |
f_open(fil_id, full_name, mode) |
args: full_name: <String>, file full name includes path. mode: <Number>, open mode 1: Write mode, Open/Create file, If the file is existing, it will be truncated and overwritten. 2: Open file for both Read and Write. return: <Number>, result code of lvgl file system. |
Open file method, after file open, you can access file by the fil_id. The f_open() will close previous opened fil_id, if you don’t invoke f_close() before. |
f_close(fil_id) |
args: return: none |
Close file. Closes an open file object. If the file has been changed, the cached information of the file is written back to the SD Card. After close file, The fil_id will become invalid. |
f_gets(fil_id, max_len) |
args: return: <String> |
Reads a string from the file. If something wrong or reach end of file(EOF), this will return NONE. |
f_lseek(fil_id, pos) |
args: return: <Number> |
Seek file position. |
f_puts(fil_id, string) |
args: string: <String>, string to write to file return: <Number>, wrote length. |
Write a string to the file. |
f_reads(fil_id, max_len) |
args: return: <String> |
This method just like f_gets() but this will add 2 char at begining of return string. 1st char represent the result code: 2nd char is a delimiter '|' A read OK example may like this: When file read reach EOF it will return If no SD Cards exist it will return Use this method can help to identify if the file has reached the EOF or just get an empty string. |
f_size(fil_id) |
args: return: <Number>, file size in bytes. |
Get file size |
f_skips(fil_id, nline) |
args: return: <Number>, skip lines |
For reading a file, skip n line from current position and return actual skipped line count. |
f_tell(fil_id) |
args: return: <Number> |
Get file pointer position. |
9. Real Time Clock (RTC)
The Real Time Clock (RTC) controller provides the real time clock and calendar information. (this feature is only available for models with RTC).
9.1. RTC API in sys object
RTC API is included in sys object. So you can invoke RTC API like
/* Set current time to 2020-12-31 23:30:0 */
sys.rtc(2020, 12, 31, 23, 30, 0);
Method |
arguments & return |
Desc. |
rtc(year, month, day, hour, min, sec) |
args: return: None |
Set current time of RTC. |
rtc(field) |
args: field, <Number>, index of RTC field return: <Number>, the value of RTC field |
Get field value (ie: year, month, day, hour, min, sec) of RTC.
|
rtc_str(format) |
args: format, <Number>, string format of RTC return: <String>, string of RTC time |
Get a formated string of RTC time
|
10. Wav File playback
BunHMI can play wavfile from SDCard (this feature is only available for models with audio output). The wav file format supported are
-
Channels: 1 or 2
-
Sampling bits: 8/16/24 bits
10.1. API in sys object
Wav play API is included in sys object. So you can invoke wav play API like
/* Play wav file */
sys.wav_play("file.wav");
Method |
arguments & return |
Desc. |
wav_play(file, [pause=0], [loop=0]) |
args: If pass file argument only, the pause and loop arguments are 0. return: <Number>
0:OK |
Open and Play wav file in SD Card, If you want set audio volume value at beginning, you can set pause=1, and adjust audio volume, then disable pause to start play audio. When the wav file read error or to the end. BunHMI will send string !^WAV_PLAY_END to notify MCU that wav play run to the end. |
wav_pause(pause) |
args: return: none |
Pause/Unpause wav playing |
wav_stop() |
args: none return: none |
Stop wav playing |
wav_dur() |
args: none return: <Number>, duration of wav file in 0.01 Sec. |
Get duration of current playing wav file. |
wav_cur() |
args: none return: <Number>, current playing time of wav file in 0.01 Sec. |
Get current playing time of wav file. |
wav_to(time) |
args: time: <Number>, Set playing wav file to time, in 0.01 Sec. return: none |
Set playing wav file to time |
audio_mute(mute) |
args: mute: <Number>, 1: Audio mute, 0: Audio unmute. return: none |
Mute/Unmute audio |
audio_vol(l_vol, r_ovl) |
args: return: none |
Set audio output volume. |
audio_vol() |
args: none return: <String>: left_vol,right_vol |
Get audio output volume. |
11. Buntalk with Checksum
When using Buntalk communication there are chance with bit error on UART. You can use checksum to check if there are bit error occure.
11.1. Checksum format
The checksum calculation is to sum of all char’s of Buntalk script and modulo by 0xff, and append 2 hex number at the end of script.
When using checksum format, you must add <ETB>(17h) at the end of command string. instead of <EOT>(04h). BunHMI will check last byte of script, when last byte of script is <ETB>(17h), it will check script with checksum.
Here is an example, if you want send:
ptr("Hello");
Then sum up all chars.
70h + 74h + 72h + 28h + 22h + 48h + 65h + 6Ch + 6Ch + 6Fh + 22h + 29h + 3Bh= 41Ah
The modulo of 41Ah is 1Ah
Then you have to send:
ptr("Hello");1A<ETB>
If checksum is error, you will get error response message
!!cksum err<EOT>
11.2. Print with checksum
When you want receive string from UART with checksum, you can use ptk() function. Just like ptr(), but ptk() will append 2 hex number of checksum at the end of string.
For example, when run script
ptk("Buntalk");
The sum of "Buntalk":
42h + 75h + 6Eh + 74h + 61h + 6Ch + 6Bh = 2D1h
The modulo of 2D1h is D1h, So you will get string
BuntalkD1<ETB>
You can ckeck last byte first, if last byte is <ETB>(17h), then last two chars before <ETB> is the checksum.